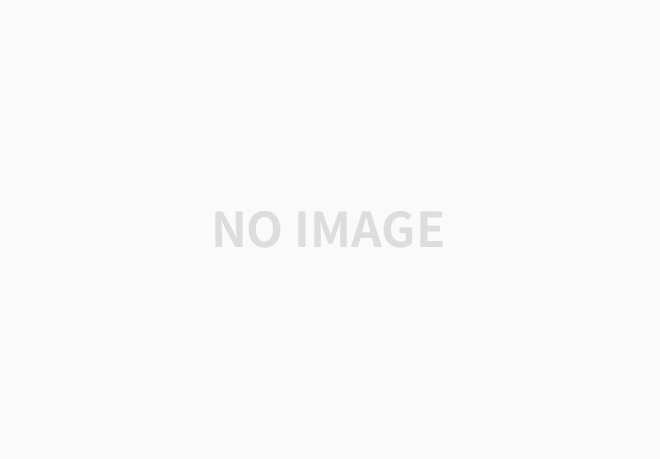
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="15dp"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center">
<ImageView
android:layout_width="150dp"
android:layout_height="150dp"
android:src="@drawable/pepper" />
</LinearLayout>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="15dp"
android:text="아이디"/>
<EditText
android:id="@+id/idEdt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="이메일 양식으로 입력"
android:inputType="textEmailAddress"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="15dp"
android:text="비밀번호"/>
<EditText
android:id="@+id/pwEdt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="비밀번호 입력"
android:inputType="textPassword"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1" />
<Button
android:id="@+id/loginBtn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="로그인"/>
</LinearLayout>
package com.gdh.loginlogictest
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Toast
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
loginBtn.setOnClickListener {
// 로그인 버튼이 눌렸을 때
// 아이디, 비번에 뭐라고 적혀있는지 각각 변수에 저장
// 아이디: admin@test.com AND 비번: qwer 질문(검사)
val inputId = idEdt.text.toString()
val inputPw = pwEdt.text.toString()
if (inputId == "admin@test.com" && inputPw == "qwer"){
Toast.makeText(this,"관리자 로그인입니다",Toast.LENGTH_SHORT).show()
}else{
Toast.makeText(this,"로그인에 실패하셨습니다",Toast.LENGTH_SHORT).show()
}
}
}
}
데이터를 받아와야 하는 태그에 id를 부여해준다. 그리고 kotlin에서 setOnclickListener을 사용하여 로그인 버튼이 눌렸을 때, 어떤 동작을 할 것인지 작성해준다. 새로운 변수를 선언하여 가져온 데이터를 넣어준다.
관리자 로그인인지 확인하기 위해 간단히 if문을 작성한다. 로그인에 성공 여부를 Toast를 이용하여 사용자에게 알려준다.
'공부 > Kotlin' 카테고리의 다른 글
[안드로이드] Android SDK 라이센스 빌드 오류 (0) | 2021.04.18 |
---|---|
[안드로이드] intent 연습 (0) | 2020.08.28 |
[안드로이드] 토스트(Toast) (0) | 2020.08.28 |
[안드로이드] 버튼 이벤트 처리 - setOnClickListener (0) | 2020.08.28 |
[안드로이드] Kotlin 기초 (0) | 2020.08.28 |